Introduction
In this multi-part article, I want to show you how you can start building WordPress Gutenberg sidebar plugins by developing a simple SEO tag editor sidebar called “Metatags” as shown below.
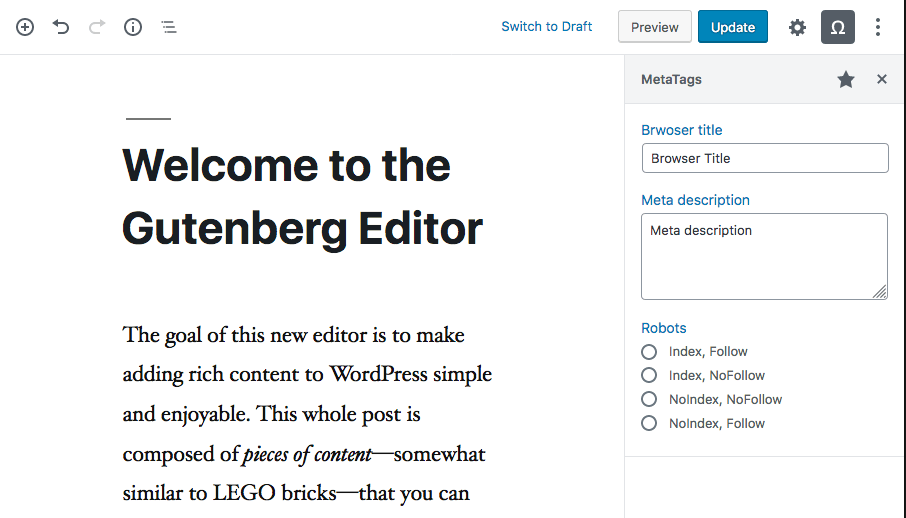
I know… it doesn’t look very fancy, but it will serve well in covering the core principles of developing sidebar plugins. Plus aesthetics are not really the point of this article.
Finished reference project
I created a GitHub Repository of the finished project as a reference for these articles. You can download and activate it in a (local) WordPress install if you want to check it out.
Registering the plugin with (Oop) Php
In this first article we will be dealing with registering the plugin with WordPress using a Php (singleton) class. if you are not familiar with using Oop in Php I can really recommend reading up on some of it’s principles but you should be able to follow along.
To get started, like with any WordPress plugin, we are going to need a fresh WordPress install, and we need to create a new folder within /wp-content/plugins. For this project we are going to name this folder “metatags”.
Inside our new metatags folder we need to create a new file called metatags.php. This file will be auto detected by WordPress and it will serve as our plugins entry-point.
To get our plugin started paste the code shown below into the new metatags.php
There are a couple of things going on here. so lets walk through the steps.
- At the top we have our standard plugin header telling WordPress about our plugin.
- Next we define a Php namespace to protect our code from name collisions.
- We then check if our plugin file is not being loaded directly, outside of the WordPress context.
- And finally we define our MetaTags class.
Adding some class properties
Next we will need to add some properties to our class. Copy the code shown below to the MetaTags class.
The properties we define here are:
- $instance: Will store a single instance of our class.
- $pluginslug: The name/text-domain of our plugin
- $metafields: An array of meta tag fields.
- $dependencies: The Gutenberg packages our plugin will be depending on.
At this point it may not be clear what these properties are for, but we’ll get to them later.
Constructor method
In our case we don’t really need a constructor method, but it’s my personal preference to add an empty one anyway. Just in case i need it later.
You can copy the constructor method into our class below the properties we defined earlier.
Singleton instance method
Next up is the instance method. Copy and paste the following method into our class below the constructor method.
This instance method makes sure that there will only be one instance of our class, by first checking if the $instance property already contains an instance of our class. If not it will create one and return that instance, else it will simply return the instance that was created earlier.
Note that we define this method as being static. This means we can call this method on our class without the need to create an instance using the new keyword. Since it’s this method’s job to create the instance, creating an instance first would be pretty redundant!
Register method
To make our plugin do something functional we are gonna need to hook into WordPress. To do so we are going to add an register method which will be responsible for adding filters and actions. Copy the following empty method to our class below the instance method.
We are going to leave this method empty for now, but we’ll add some actions later. First let’s see if we can call our class.
Calling the plugin class
To start of our class, and our plugin, we are gonna need to create a new instance. Add the following code, below our class.
Here we create a new function called runMetaTags. this function calls the static instance method on the MetaTags class. The instance method will return an instance of the class. We then immediately call the register method on the new instance. Later on the register method will register our actions.
At this point our plugin doesn’t do very much, but we can check to see if WordPress is detecting our plugin, and if we can active it already.
Plugin activation
To see if our plugin is working thus far, we can go to our WordPress admin, and go to the plugins page. You should now see our plugin like shown below.

You can now activate the plugin. And if there are no errors showing we can continue to add some actions.
Adding some actions
We can now start to add some functionality to our plugin by adding a couple of actions. Edit the register method of our class to reflect the version shown below.
Here we use the add_actions function to add three actions to our plugin.
We add two actions to the enqueue_block_editor_assets hook. One for adding Css files (the enqueue_styles method) and one for adding Javascript files (the enqueue_scripts method). We could add both file types with one action, but i like to keep things neatly separated into there own methods.
Note that we are using the enqueue_block_editor_assets hook instead of the admin_enqueue_scripts hook. This will make sure that our files will only be added if the Block editor is present, so we don’t have to check if we are on the right page, or which editor is being used.
We then add a third action to the init hook, and add the register_meta_fields method to it. This method will be responsible for registering custom meta fields with WordPress.
Also note the use of the backslash before the add_actions function. Because our class is in its own namespace Php will try to find the add_action function within that namespace, if it can’t find it, only then will it look in the global namespace. Adding the backslash tells Php that we mean the function in the global namespace. So it can skip checking the current namespace speeding up execution.
Enqueue styles method
Next up is the enqueue_styles method. add this method below the register method in our class.
With this method we use the wp_enqueue_style function to add a Stylesheet to the Gutenberg editor. This piece of code should look familiar. But do note that we use the $this->pluginslug property, we added earlier, to make a name spaced handle for our Stylesheet, since this has to be a unique value.
To test if our Stylesheet is being added correctly we can create a file dist/css/metatags.css in out theme folder. and add the following content to this new file.
If you save the class and the new Stylesheet, and go to any post editor screen that uses the Gutenberg editor, the page should now look all weird and have red borders around all the elements meaning our styles are being loaded.
If not, go and reference the GitHub repository of the finished project, or the completed class at the end of this article, to see if you missed something along the way.
Enqueue scripts method
Besides a Stylesheet we are also gonna need to add a Javascript file which will contain our React code for the sidebar plugin. Add the enqueue_scripts method shown below to our class after the enqueue_styles method.
This method is almost identical to the previous one, except that we use the wp_enqueue_scripts function and pass the $this->dependencies property to the dependencies parameter. This property contains a list of Gutenberg packages that out plugin will depend on, and that need to be loaded before our script can run.
To test if this file is being loaded correctly create a new file dist/js/metatags.js in our theme folder. and add the code below.
Save the files and refresh the edit page in the WordPress admin. Besides looking all funky with red borders it should now also give us an alert message.
Again, if you don’t see the alert go and reference the github repo of the finished project, or the completed class at the end of this article, to see if you missed something along the way.
Register meta fields method
Before we can start with the Javascript code there is one more Php thing we need to take care of.
Our plugin will use a couple of post meta fields/values, a.k.a. custom fields, to store our SEO meta data. These fields have to be registered with WordPress before we can access them in the editor.
So let’s take care of that. Add the register_meta_fields method shown below to our class after the enqueue_scripts method.
With the register_meta_fields method we first create a array of settings that we will pass for all of our fields. Then we loop over the $this->metafields property and register each of them with WordPress using the register_meta function.
With this method we should now be able to access these fields from within the Gutenberg editor.
Semi completed plugin class
That’s it for now on the Php side of our plugin. Below you’ll find the semi completed plugin class complemented with some extra comments to keep things clear as we move along. You can also check out the github repo of the finished project.
Within the comments in the code below you can find references to the docs of the WordPress functions being used. These functions are often used when building plugins so i recommend you take a second to check out these docs.
Moving forward
That’s it for now setting up our plugin with Php. We will come back to this class later to add our meta field data to the head of our theme as Meta tags. In the next article we will add a folder structure and the Laravel Mix Webpack wrapper to bundle our Css and Javascript assets.
Follow me on twitter @Vanaf1979 or on Dev.to @Vanaf1979 to be notified about the next article in this series, and other WordPress related stuff.
If you have any questions, want to leave a comment or want to point out a mistake i made please visit the copy of this article on Dev.to and drop you comment there so i can get back to you.
Thanks for reading.